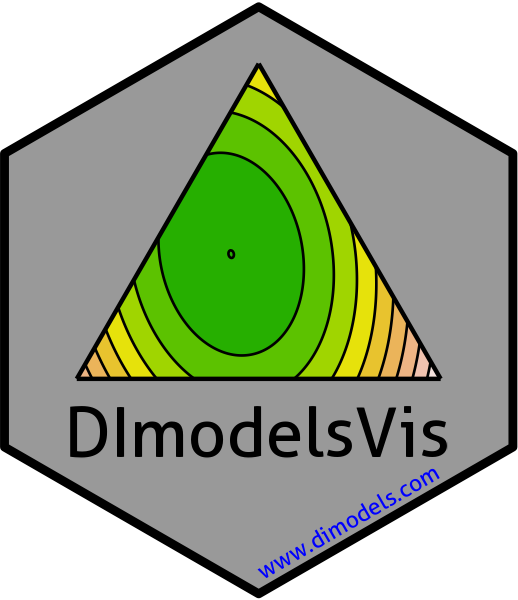
Copy attributes from one object to another
copy_attributes.Rd
This function copies over any additional attributes from `source`
into `target`. Any attributes already present in `target` would be
left untouched. This function is useful after manipulating the data
from the *_data
preparation functions to ensure any attributes
necessary for creating the plot aren't lost.
Examples
## Simple example
a <- data.frame(Var1 = runif(1:10), Var2 = runif(1:10))
b <- data.frame(Var3 = runif(1:10), Var4 = runif(1:10))
attr(b, "attr1") <- "Lorem"
attr(b, "attr2") <- "ipsum"
print(attributes(a))
#> $names
#> [1] "Var1" "Var2"
#>
#> $class
#> [1] "data.frame"
#>
#> $row.names
#> [1] 1 2 3 4 5 6 7 8 9 10
#>
print(attributes(b))
#> $names
#> [1] "Var3" "Var4"
#>
#> $class
#> [1] "data.frame"
#>
#> $row.names
#> [1] 1 2 3 4 5 6 7 8 9 10
#>
#> $attr1
#> [1] "Lorem"
#>
#> $attr2
#> [1] "ipsum"
#>
## Copy over attributes of `b` into `a`
print(copy_attributes(target = a, source = b))
#> Var1 Var2
#> 1 0.67838043 0.2255625
#> 2 0.73531960 0.3008308
#> 3 0.19595673 0.6364656
#> 4 0.98053967 0.4790245
#> 5 0.74152153 0.4321713
#> 6 0.05144628 0.7064338
#> 7 0.53021246 0.9485766
#> 8 0.69582388 0.1803388
#> 9 0.68855600 0.2168999
#> 10 0.03123033 0.6801629
## Note the attributes already present in `a` are left untouched
## Can also be used in the dplyr pipeline
library(dplyr)
iris_sub <- iris[1:10, ]
attr(iris_sub, "attr1") <- "Lorem"
attr(iris_sub, "attr2") <- "ipsum"
attributes(iris_sub)
#> $names
#> [1] "Sepal.Length" "Sepal.Width" "Petal.Length" "Petal.Width" "Species"
#>
#> $row.names
#> [1] 1 2 3 4 5 6 7 8 9 10
#>
#> $class
#> [1] "data.frame"
#>
#> $attr1
#> [1] "Lorem"
#>
#> $attr2
#> [1] "ipsum"
#>
## Grouping can drop attributes we set
iris_sub %>%
group_by(Species) %>%
summarise(mean(Sepal.Length)) %>%
attributes()
#> $class
#> [1] "tbl_df" "tbl" "data.frame"
#>
#> $row.names
#> [1] 1
#>
#> $names
#> [1] "Species" "mean(Sepal.Length)"
#>
## Use copy_attributes with `iris_sub` object as source
## to add the attributes again
iris_sub %>%
group_by(Species) %>%
summarise(mean(Sepal.Length)) %>%
copy_attributes(source = iris_sub) %>%
attributes()
#> $class
#> [1] "tbl_df" "tbl" "data.frame"
#>
#> $row.names
#> [1] 1
#>
#> $names
#> [1] "Species" "mean(Sepal.Length)"
#>
#> $attr1
#> [1] "Lorem"
#>
#> $attr2
#> [1] "ipsum"
#>