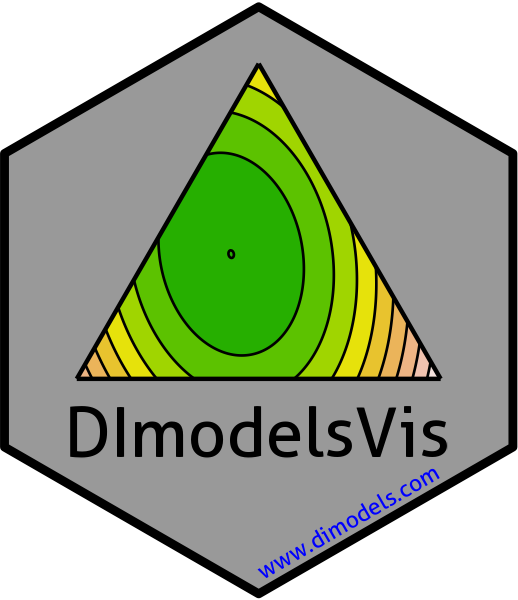
Add identity effect groups used in a Diversity-Interactions (DI) model to new data
add_ID_terms.Rd
Utility function that accepts a fitted Diversity-Interactions (DI) model object along with a data frame and adds the appropriate species identity effect groupings to the data for making predictions.
Arguments
- data
A data-frame with species proportions that sum to 1 to create the identity effect groupings.
- model
A Diversity Interactions model object fit using the
DI()
orautoDI()
functions from theDImodels
orDImulti()
from theDImodelsMulti
R packages.
Value
A data-frame with additional columns appended to the end that contain the grouped species proportions.
Examples
library(DImodels)
data(sim1)
# Fit DI models with different ID effect groupings
mod1 <- DI(y = "response", prop = 3:6,
data = sim1, DImodel = "AV") # No ID grouping
#> Fitted model: Average interactions 'AV' DImodel
mod2 <- DI(y = "response", prop = 3:6,
data = sim1, DImodel = "AV",
ID = c("ID1", "ID1", "ID2", "ID2"))
#> Fitted model: Average interactions 'AV' DImodel
mod3 <- DI(y = "response", prop = 3:6,
data = sim1, DImodel = "AV",
ID = c("ID1", "ID1", "ID1", "ID1"))
#> Fitted model: Average interactions 'AV' DImodel
# Create new data for adding interaction terms
newdata <- sim1[sim1$block == 1, 3:6]
print(head(newdata))
#> p1 p2 p3 p4
#> 1 0.70 0.10 0.10 0.10
#> 5 0.10 0.70 0.10 0.10
#> 9 0.10 0.10 0.70 0.10
#> 13 0.10 0.10 0.10 0.70
#> 17 0.25 0.25 0.25 0.25
#> 21 0.40 0.40 0.10 0.10
add_ID_terms(data = newdata, model = mod1)
#> p1 p2 p3 p4 p1_ID p2_ID p3_ID p4_ID
#> 1 0.70 0.10 0.10 0.10 0.70 0.10 0.10 0.10
#> 5 0.10 0.70 0.10 0.10 0.10 0.70 0.10 0.10
#> 9 0.10 0.10 0.70 0.10 0.10 0.10 0.70 0.10
#> 13 0.10 0.10 0.10 0.70 0.10 0.10 0.10 0.70
#> 17 0.25 0.25 0.25 0.25 0.25 0.25 0.25 0.25
#> 21 0.40 0.40 0.10 0.10 0.40 0.40 0.10 0.10
#> 25 0.40 0.10 0.40 0.10 0.40 0.10 0.40 0.10
#> 29 0.40 0.10 0.10 0.40 0.40 0.10 0.10 0.40
#> 33 0.10 0.40 0.40 0.10 0.10 0.40 0.40 0.10
#> 37 0.10 0.40 0.10 0.40 0.10 0.40 0.10 0.40
#> 41 0.10 0.10 0.40 0.40 0.10 0.10 0.40 0.40
#> 45 1.00 0.00 0.00 0.00 1.00 0.00 0.00 0.00
#> 49 0.00 1.00 0.00 0.00 0.00 1.00 0.00 0.00
#> 53 0.00 0.00 1.00 0.00 0.00 0.00 1.00 0.00
#> 57 0.00 0.00 0.00 1.00 0.00 0.00 0.00 1.00
add_ID_terms(data = newdata, model = mod2)
#> p1 p2 p3 p4 ID1 ID2
#> 1 0.70 0.10 0.10 0.10 0.8 0.2
#> 5 0.10 0.70 0.10 0.10 0.8 0.2
#> 9 0.10 0.10 0.70 0.10 0.2 0.8
#> 13 0.10 0.10 0.10 0.70 0.2 0.8
#> 17 0.25 0.25 0.25 0.25 0.5 0.5
#> 21 0.40 0.40 0.10 0.10 0.8 0.2
#> 25 0.40 0.10 0.40 0.10 0.5 0.5
#> 29 0.40 0.10 0.10 0.40 0.5 0.5
#> 33 0.10 0.40 0.40 0.10 0.5 0.5
#> 37 0.10 0.40 0.10 0.40 0.5 0.5
#> 41 0.10 0.10 0.40 0.40 0.2 0.8
#> 45 1.00 0.00 0.00 0.00 1.0 0.0
#> 49 0.00 1.00 0.00 0.00 1.0 0.0
#> 53 0.00 0.00 1.00 0.00 0.0 1.0
#> 57 0.00 0.00 0.00 1.00 0.0 1.0
add_ID_terms(data = newdata, model = mod3)
#> p1 p2 p3 p4 ID1
#> 1 0.70 0.10 0.10 0.10 1
#> 5 0.10 0.70 0.10 0.10 1
#> 9 0.10 0.10 0.70 0.10 1
#> 13 0.10 0.10 0.10 0.70 1
#> 17 0.25 0.25 0.25 0.25 1
#> 21 0.40 0.40 0.10 0.10 1
#> 25 0.40 0.10 0.40 0.10 1
#> 29 0.40 0.10 0.10 0.40 1
#> 33 0.10 0.40 0.40 0.10 1
#> 37 0.10 0.40 0.10 0.40 1
#> 41 0.10 0.10 0.40 0.40 1
#> 45 1.00 0.00 0.00 0.00 1
#> 49 0.00 1.00 0.00 0.00 1
#> 53 0.00 0.00 1.00 0.00 1
#> 57 0.00 0.00 0.00 1.00 1