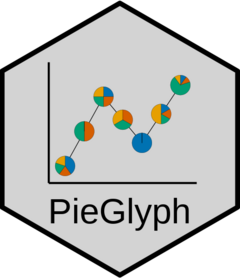
Scatter plots with interactive pie-chart glyphs
Source:R/geom_pie_interactive.R
geom_pie_interactive.Rd
This geom is based on geom_pie_glyph and replaces points in a scatter plot
with interactive pie-chart glyphs to show the relative proportions of different
categories. Like geom_pie_glyph, the pie-chart glyphs are independent of the
axes, with the additional feature of being interactive and can be hovered over
to show information about the raw counts of the different categories. The
interactivity is added using the ggiraph
framework and all features offered by ggiraph
are supported.
Arguments
- ...
arguments passed to
geom_pie_glyph
, in addition to allinteractive_parameters
offered byggiraph
.
Examples
#' ## Load libraries
library(dplyr)
library(tidyr)
library(ggplot2)
library(ggiraph)
#> Warning: package 'ggiraph' was built under R version 4.3.3
## Simulate raw data
set.seed(123)
plot_data <- data.frame(response = rnorm(10, 100, 30),
system = as.factor(1:10),
group = sample(size = 10,
x = c("G1", "G2", "G3"),
replace = TRUE),
A = round(runif(10, 3, 9), 2),
B = round(runif(10, 1, 5), 2),
C = round(runif(10, 3, 7), 2),
D = round(runif(10, 1, 9), 2))
head(plot_data)
#> response system group A B C D
#> 1 83.18573 1 G1 7.14 2.65 6.20 4.07
#> 2 93.09468 2 G1 7.77 2.48 3.49 3.20
#> 3 146.76125 3 G1 3.15 1.61 5.24 7.52
#> 4 102.11525 4 G1 5.87 1.56 3.83 4.59
#> 5 103.87863 5 G3 7.55 1.93 3.51 7.48
#> 6 151.45195 6 G2 4.30 2.86 6.01 7.50
# One of the interactive aesthetics is tooltip. It is set that by default
# it shows the value and percentage of each slice in the pie-chart.
# Hover over any pie-chart in the plot to see this
plot_obj1 <- ggplot(data = plot_data, aes(x = system, y = response)) +
geom_pie_interactive(slices = c("A", "B", "C", "D"),
data = plot_data)+
theme_classic()
x1 <- girafe(ggobj = plot_obj1)
if(interactive()) print(x1)
# The user can also set their own custom tooltip which could either by
# a column in the data or a custom string
plot_obj2 <- ggplot(data = plot_data, aes(x = system, y = response)) +
# Setting the group as a tooltip
geom_pie_interactive(slices = c("A", "B", "C", "D"),
data = plot_data,
aes(tooltip = paste0("Group: ", group)))+
theme_classic()
x2 <- girafe(ggobj = plot_obj2)
if(interactive()) print(x2)
# It is also possible to add an identifier to highlight all elements within
# a group when one element of a group is hovered over by using data_id
plot_obj3 <- ggplot(data = plot_data, aes(x = system, y = response)) +
# Setting the group as a tooltip
geom_pie_interactive(slices = c("A", "B", "C", "D"),
data = plot_data, colour = "black",
aes(data_id = group))+
theme_classic()
x3 <- girafe(ggobj = plot_obj3)
# Hover over one pie-glyph to highlight all observations within the same group
if(interactive()) print(x3)
# All other aesthetics and attributes of geom_pie_glyph can be used as well